Advisory boards aren’t only for executives. Join the LogRocket Content Advisory Board today →
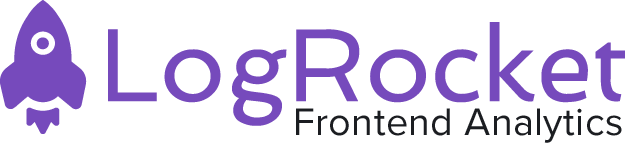
- Product Management
- Solve User-Reported Issues
- Find Issues Faster
- Optimize Conversion and Adoption
- Start Monitoring for Free

The ultimate guide to enabling Cross-Origin Resource Sharing (CORS)
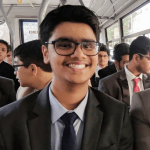
Consider the following situation: you’re trying to fetch some data from an API on your website using fetch() but end up with an error.
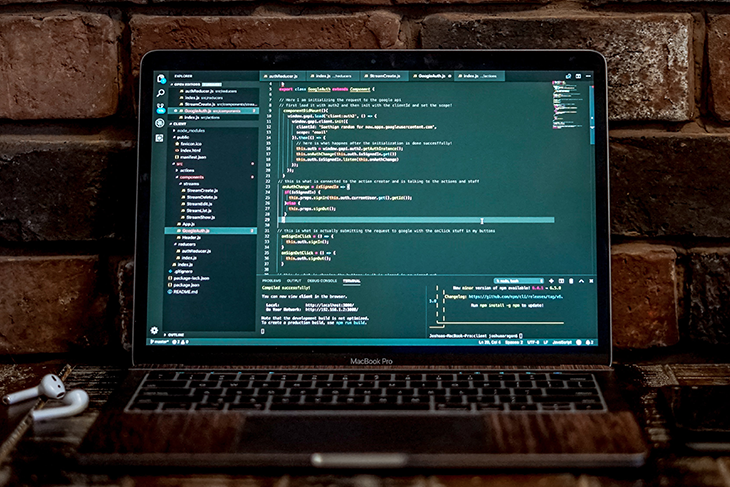
You open up the console and see either “No Access-Control-Allow-Origin header is present on the requested resource,” or “The Access-Control-Allow-Origin header has a value <some_url> that is not equal to the supplied origin” written in red text, indicating that your request was blocked by CORS policy.

Seem familiar? With over 10,000 questions posted under the cors tag on StackOverflow, it is one of the most common issues that plague frontend developers and backend developers alike. So, what exactly is the CORS policy and why do we face this error often?
What is Cross-Origin Resource Sharing (CORS)?
Interestingly, this is not an error as we portray it, but rather the expected behavior. Our web browsers enforce the same-origin policy , which restricts resource sharing across different origins. Cross-origin resource sharing , or CORS, is the mechanism through which we can overcome this barrier. To understand CORS, let us first understand the same-origin policy and its need.
The same-origin policy
In simple terms, the same-origin policy is the web version of “don’t talk to strangers” incorporated by the browser.
All modern web browsers available today follow the same-origin policy that restricts how XMLHttpRequest and fetch requests from one origin interact with a resource from another origin. What’s an origin, exactly?
It’s the combination of a scheme, domain, and port. The scheme could be HTTP, HTTPS, FTP, or anything else. Similarly, the port can also be any valid port number. Same-origin requests are essentially those requests whose scheme, domain, and port match. Let’s look at the following example.
Assuming our origin is http://localhost:3000 , the requests can be categorized into same-origin or cross-origin requests as follows:
This is the reason why your frontend running on http://localhost:3000 cannot make API calls to your server running http://localhost:5000 or any other port when you develop single-page applications (SPAs).
Also, requests from origin https://mywebsite.com to origin https://api.mywebsite.com are still considered cross-site requests even though the second origin is a subdomain.
Due to the same-origin policy, the browser will automatically prevent responses from cross-origin requests from being shared with the client. This is great for security reasons! But not all websites are malicious and there are multiple scenarios in which you might need to fetch data from different origins, especially in the modern age of microservice architecture where different applications are hosted on different origins.
This is a great segue for us to deep dive into CORS and learn how to use it in order to allow cross-origin requests.
Allowing cross-site requests with CORS
We’ve established that the browser doesn’t allow resource sharing between different origins, yet there are countless examples where we are able to do so. How? This is where CORS comes into the picture.
CORS is an HTTP header-based protocol that enables resource sharing between different origins. Alongside the HTTP headers, CORS also relies on the browser’s preflight-flight request using the OPTIONS method for non-simple requests. More on simple and preflight requests later in this article.
Because HTTP headers are the crux of the CORS mechanism, let’s look at these headers and what each of them signifies.
Access-Control-Allow-Origin
The Access-Control-Allow-Origin response header is perhaps the most important HTTP header set by the CORS mechanism. The value of this header consists of origins that are allowed to access the resources. If this header is not present in the response headers, it means that CORS has not been set up on the server.
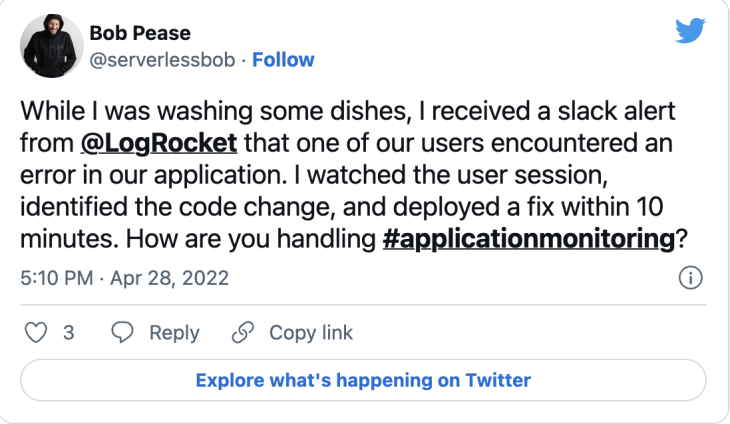
Over 200k developers use LogRocket to create better digital experiences

If this header is present, its value is checked against the Origin header of request headers. If the values match, the request will be completed successfully and resources will be shared. Upon mismatch, the browser will respond with a CORS error.
To allow all origins to access the resources in the case of a public API, the Access-Control-Allow-Origin header can be set to * on the server. In order to restrict only particular origins to access the resources, the header can be set to the complete domain of the client origin such as https://mywebsite.com .
Access-Control-Allow-Methods
The Access-Control-Allow-Methods response header is used to specify the allowed HTTP method or a list of HTTP methods such as GET , POST , and PUT that the server can respond to.
This header is present in the response to pre-flighted requests. If the HTTP method of your request is not present in this list of allowed methods, it will result in a CORS error. This is highly useful when you want to restrict users from modifying the data through POST , PUT , PATCH , or DELETE requests.
Access-Control-Allow-Headers
The Access-Control-Allow-Headers response header indicates the list of allowed HTTP headers that your request can have. To support custom headers such as x-auth-token , you can set up CORS on your server accordingly.
Requests that consist of other headers apart from the allowed headers will result in a CORS error. Similar to the Access-Control-Allow-Methods header, this header is used in response to pre-flighted requests.
Access-Control-Max-Age
Pre-flighted requests require the browser to first make a request to the server using the OPTIONS HTTP method. Only after this can the main request be made if it is deemed safe. However, making the OPTIONS call for each pre-flighted request can be expensive.
To prevent this, the server can respond with the Access-Control-Max-Age header, allowing the browser to cache the result of pre-flighted requests for a certain amount of time. The value of this header is the amount of time in terms of delta seconds.
More great articles from LogRocket:
- Don't miss a moment with The Replay , a curated newsletter from LogRocket
- Learn how LogRocket's Galileo cuts through the noise to proactively resolve issues in your app
- Use React's useEffect to optimize your application's performance
- Switch between multiple versions of Node
- Discover how to use the React children prop with TypeScript
- Explore creating a custom mouse cursor with CSS
- Advisory boards aren’t just for executives. Join LogRocket’s Content Advisory Board. You’ll help inform the type of content we create and get access to exclusive meetups, social accreditation, and swag.
Overall, here’s the syntax of how CORS response headers look like:
Simple requests vs. pre-flighted requests
Requests that do not trigger a CORS preflight fall under the category of simple requests. However, the request has to satisfy some conditions only after it is deemed as a simple request. These conditions are:
- The HTTP method of the request should be one of these: GET , POST , or HEAD
- The request headers should only consist of CORS safe-listed headers such as Accept , Accept-Language , Content-Language , and Content-Type apart from the headers automatically set by the user agent
- The Content-Type header should have only either of these three values: application/x-www-form-urlencoded , multipart/form-data , or text/plain
- No event listeners are registered on the object returned by the XMLHttpRequest.upload property if using XMLHttpRequest
- No ReadableStream object should be used in the request
On failing to satisfy either of these conditions, the request is considered to be a pre-flighted request. For such requests, the browser has to first send a request using the OPTIONS method to the different origin.
This is used to check if the actual request is safe to send to the server. The approval or rejection of the actual request depends on the response headers to the pre-flighted request. If there is a mismatch between these response headers and the main request’s headers, the request is not made.
Enabling CORS
Let’s consider our initial situation where we faced the CORS error. There are multiple ways we could resolve this issue depending on whether we have access to the server on which the resources are hosted. We can narrow it down to two situations:
- You have access to the backend or know the backend developer
- You can manage only the frontend and cannot access the backend server
If you have access to the backend:
Because CORS is just an HTTP header-based mechanism, you can configure the server to respond with appropriate headers in order to enable resource sharing across different origins. Have a look at the CORS headers we discussed above and set the headers accordingly.
For Node.js + Express.js developers, you can install the cors middleware from npm. Here is a snippet that uses the Express web framework, along with the CORS middleware:
If you don’t pass an object consisting of CORS configuration, the default configuration will be used, which is equivalent to:
Here is how you could configure CORS on your server which will only allow GET requests from https://yourwebsite.com with headers Content-Type and Authorization with a 10 minutes preflight cache time:
While this code is specific to Express.js and Node.js, the concept remains the same. Using the programming language and framework of your choice, you can manually set the CORS headers with your responses or create a custom middleware for the same.
If you only have access to the frontend:
Quite often, we may not have access to the backend server. For example, a public API. Due to this, we cannot add headers to the response we receive. However, we could use a proxy server that will add the CORS headers to the proxied request.
The cors-anywhere project is a Node.js reverse proxy that can allow us to do the same. The proxy server is available on https://cors-anywhere.herokuapp.com/ , but you can build your own proxy server by cloning the repository and deploying it on a free platform like Heroku or any other desired platform.
In this method, instead of directly making the request to the server like this:
Simply append the proxy server’s URL to the start of the API’s URL, like so:
As we learn to appreciate the same-origin policy for its security against cross-site forgery attacks, CORS does seem to make a lot of sense. While the occurrences of the red CORS error messages in the console aren’t going to magically disappear, you are now equipped with the knowledge to tackle these messages irrespective of whether you work on the frontend or the backend.
Get set up with LogRocket's modern error tracking in minutes:
- Visit https://logrocket.com/signup/ to get an app ID
Install LogRocket via npm or script tag. LogRocket.init() must be called client-side, not server-side
Share this:
- Click to share on Twitter (Opens in new window)
- Click to share on Reddit (Opens in new window)
- Click to share on LinkedIn (Opens in new window)
- Click to share on Facebook (Opens in new window)
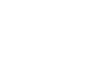
Stop guessing about your digital experience with LogRocket
Recent posts:.
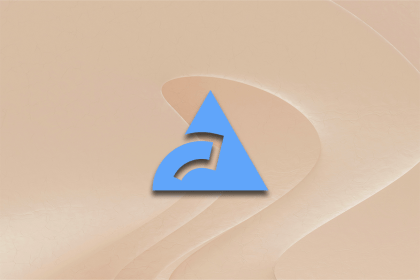
Biome adoption guide: Overview, examples, and alternatives
Biome combines linters and formatters into one tools, helping developers write better code faster and with less setup and configuration.
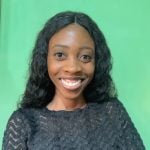
React Native layout management with Yoga 3.0
Explore layout management in your React Native apps with the latest release of React Native v0.74 and Yoga 3.0.
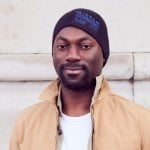
A guide to JavaScript parser generators
Explore three JavaScript parser generator libraries and the benefits of creating custom parsers for specific project needs.
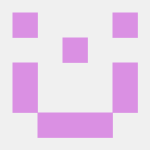
Using Rust and Axum to build a JWT authentication API
Learn to build a basic JWT authentication system with Rust and Axum, including setting up the routes, handlers, and the middleware system.
2 Replies to "The ultimate guide to enabling Cross-Origin Resource Sharing (CORS)"
In depth and finly captured.
I may have missed something but if we can kind of bypass the cors policy by just using cors-anywhere, what’s the point in using cors/setting up cors in the server anyway????
Leave a Reply Cancel reply
The Access-Control-Allow-Origin Header Explained – With a CORS Example

Often times when calling an API, you may see an error in your console that looks like this:
In this post, we are going to learn why this error happens and how you can fix it.
What is the Access-Control-Allow-Origin header?
Access-Control-Allow-Origin is a CORS header. CORS, or Cross Origin Resource Sharing, is a mechanism for browsers to let a site running at origin A to request resources from origin B.
Origin is not just the hostname, but a combination of port, hostname and scheme, such as - http://mysite.example.com:8080/
Here's an example of where this comes into action -
- I have an origin A: http://mysite.com and I want to get resources from origin B: http://yoursite.com .
- To protect your security, the browser will not let me access resources from yoursite.com and will block my request.
- In order to allow origin A to access your resources, your origin B will need to let the browser know that it is okay for me to get resources from your origin.
Here is an example from Mozilla Developer Network that explains this really well:
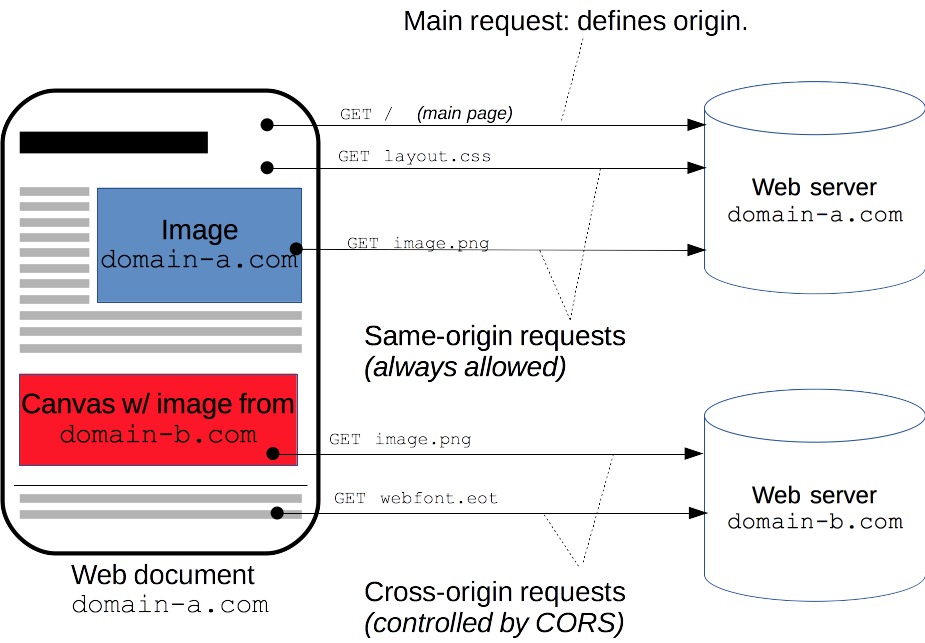
With the help of CORS, browsers allow origins to share resources amongst each other.
There are a few headers that allow sharing of resources across origins, but the main one is Access-Control-Allow-Origin . This tells the browser what origins are allowed to receive requests from this server.
Who needs to set Access-Control-Allow-Origin ?
To understand who needs to set this header, consider this scenario: You are browsing a website that is used to view and listen to songs. The website attempts to make a connection to your bank in the background maliciously.
So who has the ultimate ability to prevent this malicious website from stealing your data from the bank? The bank! So, the bank will need to protect its resources by setting the Access-Control-Allow-Origin header as part of the response.
Just remember: the origin responsible for serving resources will need to set this header.
How to use and when to pass this header
Here's an example of values you can set:
- Access-Control-Allow-Origin : * : Allows any origin.
- Access-Control-Allow-Origin : http://mysite.com : Allow requests only from mysite.com.

See it in action
Let's look at an example. You can check out this code on my GitHub repo .
We are going to build a server on origin A http://localhost:8000 which will send a string of Hello s to an api endpoint. We are going to call with this endpoint by creating a client on origin B http://localhost:3000 and then use fetch to request the resource. We expect to see the string Hello passed by origin A in the browser console of origin B.
Let's say we have an origin up on http://localhost:8000 that serves up this resource on /api endpoint. The server sends a response with the header Access-Control-Allow-Origin .
On the client side, you can call this endpoint by calling fetch like this:
Now open your browser's console to see the result. Since the header is currently set to allow access only from https://yoursite.com , the browser will block access to the resource and you will see an error in your console.

Now, to fix this, change the headers to this:
Check your browser's console and now you will be able to see the string Hello .
Interested in more tutorials and JSBytes from me? Sign up for my newsletter .
Read more posts .
If you read this far, thank the author to show them you care. Say Thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
Today I Learned
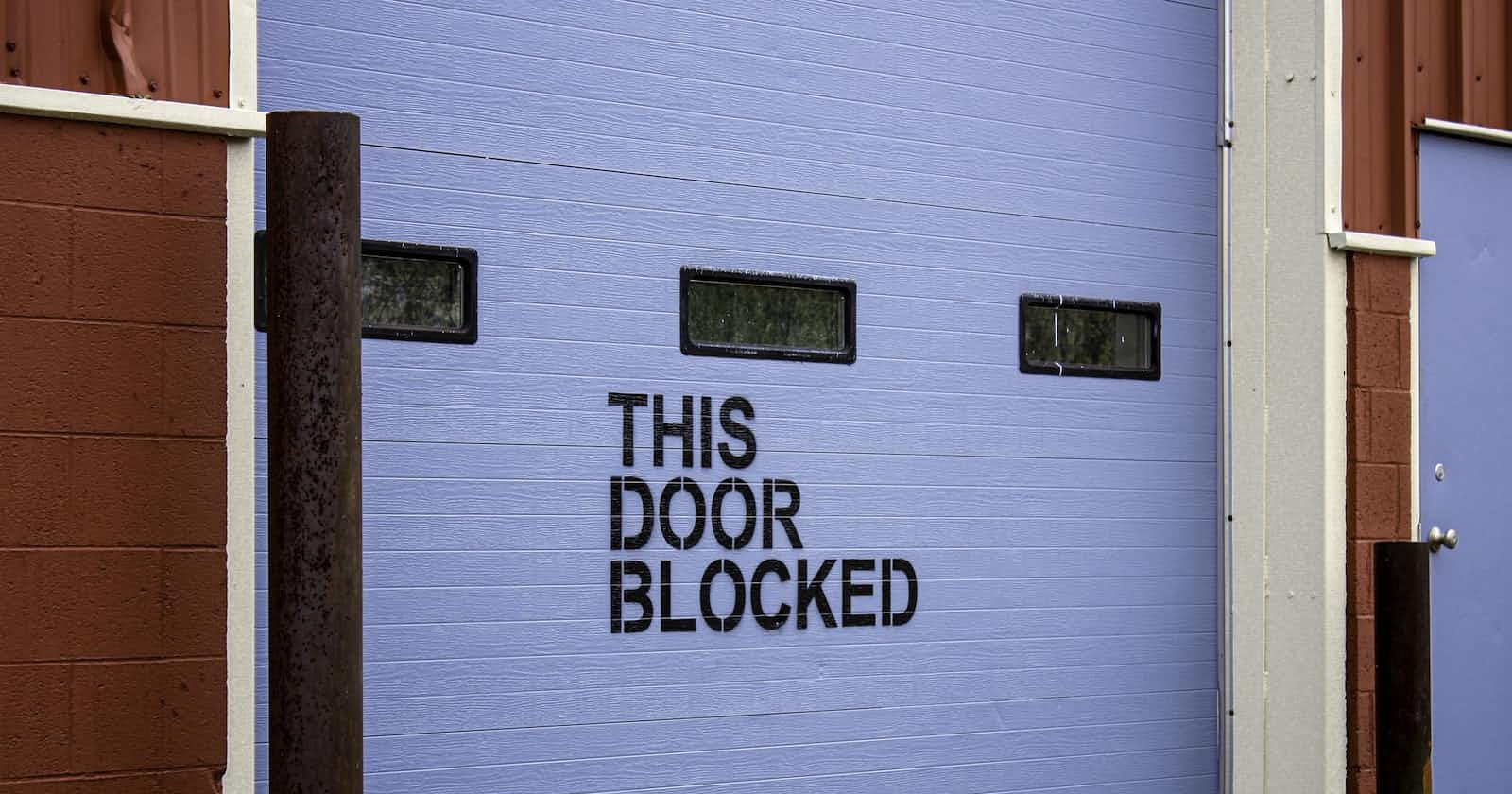
Photo by Morgane Perraud on Unsplash
Demystifying CORS: Understanding Access-Control-Allow-Origin
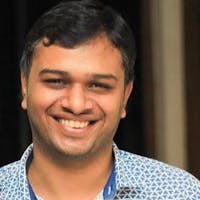
Cross-Origin Resource Sharing (CORS) is a security feature implemented by web browsers to control how web pages in one domain can request and interact with resources from another domain. CORS is crucial for maintaining a secure and controlled web environment.
In this blog post, we'll delve into one of the common CORS-related issues we encounter—the "Access-Control-Allow-Origin" error—and explore ways to address it.
Understanding the Error:
Imagine you're developing a web application on your local machine, running at ' http://localhost:3000 ', and you attempt to make an XMLHttpRequest to fetch data from ' https://example.com '. If the server at ' https://example.com ' lacks the appropriate CORS headers, the browser will block the request, triggering an error like:
Breaking Down CORS:
CORS operates on the principle of restricting web pages from making requests to a domain different from the one that served the web page. The 'Access-Control-Allow-Origin' header plays a pivotal role in CORS by indicating which origins are permitted to access the resources on the server.
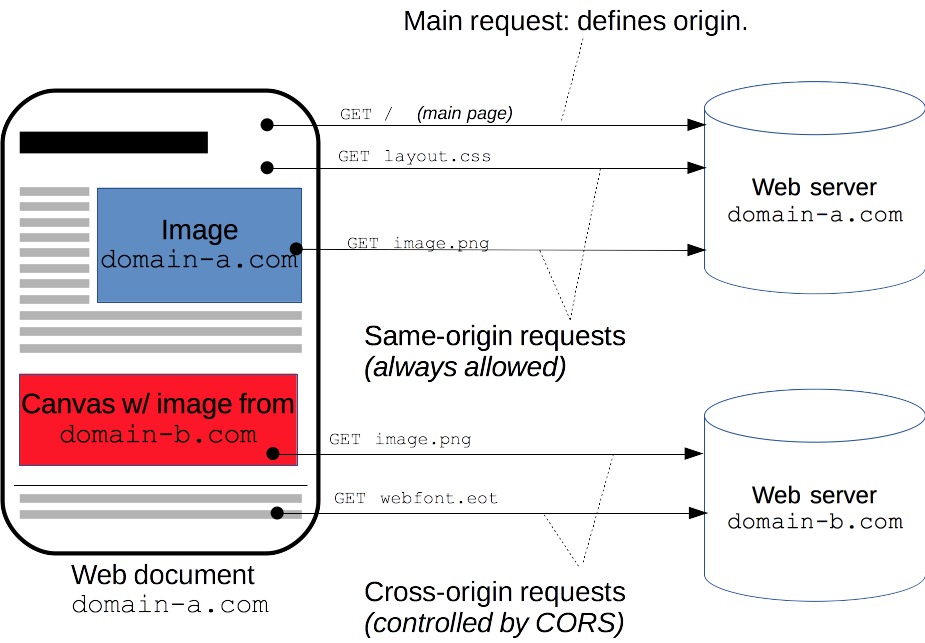
To send an OPTIONS request to a server using CURL, we can use the following command:
Explanation of the flags and headers:
-i : Includes the HTTP headers in the output.
-X 'OPTIONS' : Specifies the HTTP method as OPTIONS.
Various -H flags: Set headers for the request, including authority, accept, accept-language, access-control-request-headers, access-control-request-method, origin, referer, sec-fetch-dest, sec-fetch-mode, sec-fetch-site, and user-agent.
--compressed : Requests compressed response.
We get a response like this
Let's break down the response we received:
Explanation:
HTTP/1.1 200 OK : Indicates that the server successfully processed the OPTIONS request, and the response status is "OK" (HTTP status code 200).
Access-Control-Allow-Headers : Specifies the headers that are allowed when making the actual request. The headers include Origin, Content-Type, Accept, Authorization, User-Agent, Referer, X-Forwarded-For, Bugsnag-Api-Key, Bugsnag-Payload-Version, Bugsnag-Sent-At.
Access-Control-Allow-Methods: POST : Specifies that the server allows the POST method when accessing the resource.
Access-Control-Allow-Origin: : Indicates that any origin is allowed to access the resource. The wildcard ' ' means any origin is permitted.
Date: Fri, 29 Dec 2023 14:09:28 GMT : Provides the date and time when the response was generated.
Content-Length: 0 : Specifies the length of the response body in bytes. In this case, the response body is empty.
Via: 1.1 google : Indicates that the response passed through a Google proxy server.
Alt-Svc: h3=":443"; ma=2592000,h3-29=":443"; ma=2592000 : Specifies alternative services, including HTTP/3 and their parameters.
In summary, the server has responded with a 200 OK status, allowing the specified headers and methods for the main request. The server is configured to accept requests from any origin ( Access-Control-Allow-Origin: * ).
What are some HTTP Response Headers that we get in Options Call.
This section lists the HTTP response headers that servers return for access control requests as defined by the Cross-Origin Resource Sharing specification. The 'Access-Control-Allow-Origin' header, for instance, specifies either a single origin or uses the '*' wildcard to allow any origin to access the resource.
For example:
Vary: Origin:
The Vary HTTP response header describes the parts of the request message aside from the method and URL that influenced the content of the response it occurs in. Most often, this is used to create a cache key
If the server specifies a single origin, it should include 'Origin' in the 'Vary' response header to indicate to clients that server responses will differ based on the value of the 'Origin' request header.
Access-Control-Expose-Headers:
The 'Access-Control-Expose-Headers' header adds specified headers to the allowlist that JavaScript in browsers is allowed to access.
Access-Control-Max-Age:
The 'Access-Control-Max-Age' header indicates how long the results of a preflight request can be cached.
The delta-seconds parameter indicates the number of seconds the results can be cached.
Access-Control-Allow-Credentials:
The 'Access-Control-Allow-Credentials' header indicates whether or not the response to the request can be exposed when the credentials flag is true.
Access-Control-Allow-Methods:
The 'Access-Control-Allow-Methods' header specifies the method or methods allowed when accessing the resource, used in response to a preflight request.
Access-Control-Allow-Headers:
The 'Access-Control-Allow-Headers' header is used in response to a preflight request to indicate which HTTP headers can be used when making the actual request.
This header is the server-side response to the browser's 'Access-Control-Request-Headers' header.
The origin responsible for serving resources need to set Access-Control-Allow-Origin header.
Server-Side Configuration:
Specify Allowed Origins:
- Configure your server to include the "Access-Control-Allow-Origin" header in its responses, specifying the allowed origins.
Server-Side Frameworks:
Many server-side frameworks provide built-in support for CORS. For example, in Node.js with Express, you can use the 'cors' middleware
Local Development Configuration:
- During local development, you can disable CORS in your browser or use browser extensions to bypass it temporarily. However, this is not a recommended solution for production.
If you liked this blog, you can follow me on twitter , and learn something new with me.
AJAX Library
- Product Home
- Explore Features
- System Requirements
- Online Demo
- My Downloads
- Release History
- All Versions Download
- Getting Started
- Upgrade to v5.11
- Upgrade to v5
- Upgrade to v4
- Upgrade to v3
- Upgrade to v2
- Activating Product
- Opening Docs
- Cookies Auth
- Do I Need davX: Protocol?
- Protocol Application Detection
- Windows Install
- Linux Install
- Web Browser Extensions
- Installing Extensions Using GPO
- Configuring NTLM
- Run File Manager
- Re-branding & Building
- Troubleshooting
- Ajax Upload
- Managing Hierarchy
- Managing Properties
- Locking Items
- Cross-Origin Requests
- Class Library
- Help & Support
Cross-Origin Requests (CORS) in Internet Explorer, Firefox, Safari and Chrome
In this article.
Enabling CORS is not required for EditDocument() , DavProtocolEditDocument() and other document opening functions in DocManager . These functions engage web browser protocol application(s) that do not have CORS restrictions.
If your WebDAV server is located on a different domain, on a different port or using different protocol (HTTP / HTTPS) such requests are considered to be cross-origin requests and by default are prohibited by user agent.
Below we describe how to enable cross-origin requests in each of 4 major browsers.
In FireFox, Safari, Chrome, Edge and IE 10+
To enable cross-origin requests in FireFox, Safari, Chrome and IE 10 and later your server must attach the following headers to all responses:
These headers will enable cross-domain requests in FireFox 3.6+, Safari 4+, Chrome 4+, Edge, and IE 10+. Older versions of this browsers do not allow cross-domain requests.
Important! Firefox and Chrome require exact domain specification in Access-Control-Allow-Origin header. For servers with authentication, these browsers do not allow "*" in this header. The Access-Control-Allow-Origin header must contain the value of the Origin header passed by the client.
Optionally you can also attach the Access-Control-Max-Age header specifying the amount of seconds that the preflight request will be cached, this will reduce the amount of requests:
Important! IT Hit WebDAV Server Engine for .Net and for Java adds necessary Access-Control headers automatically. You do not need to add them manually.
In Internet Explorer 9 and Earlier
Internet Explorer 9 and earlier ignores Access-Control-Allow headers and by default prohibits cross-origin requests for Internet Zone. To enable cross-origin access go to Tools->Internet Options->Security tab, click on “Custom Level” button. Find the Miscellaneous -> Access data sources across domains setting and select “Enable” option.
If your server is located in Intranet Zane by default IE will pop the confirmation dialog during first cross-domain request: “ This page is accessing information that is not under its control. This poses a security risk. Do you want to continue? ”. To suppress this warning, you will need to set the " Access data sources across domains " setting to " Allow ".
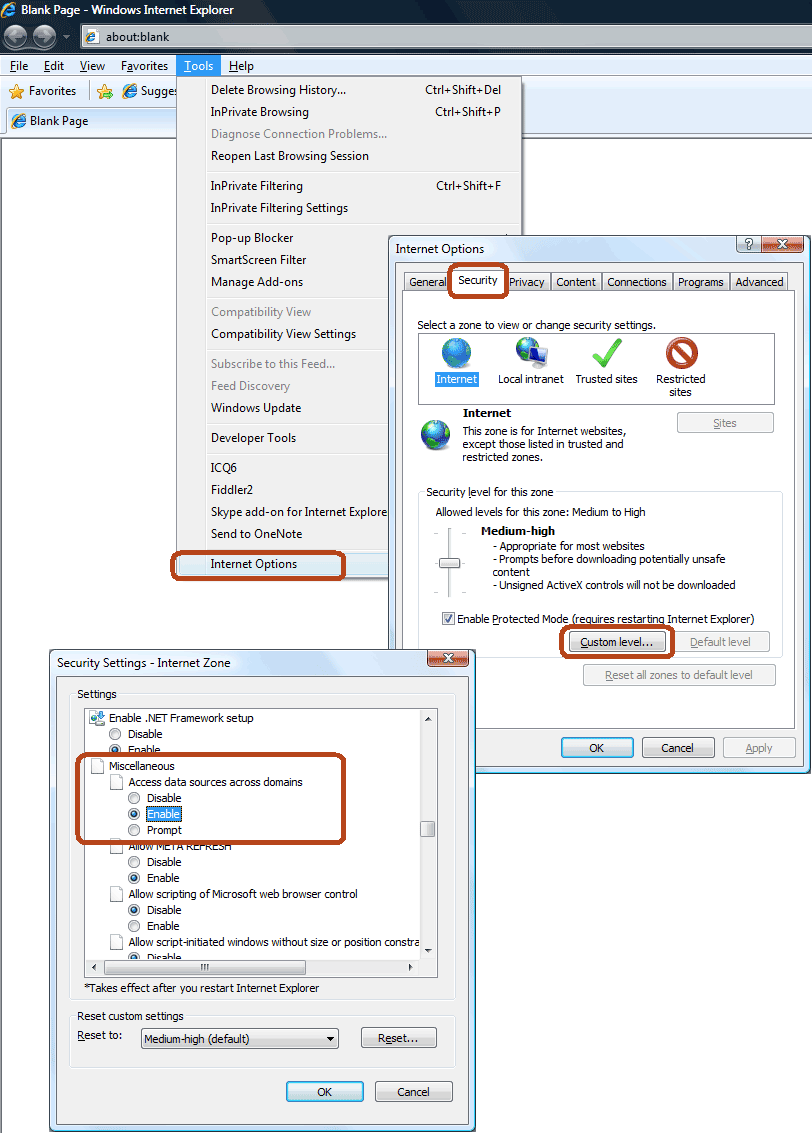
Cross-Origin Requests with Authentication
If your WebDAV server is using Basic, Digest or Integrated Windows Authentication (IWA) a user agent may imply additional limitations.
Important! - Internet Explorer on Windows 7 and Windows Vista by default require SSL connection for Basic authentication. Details about how to overcome this limitation could be found here . - Firefox requires OPTIONS request to be unauthenticated when cross-domain requests are used. - Web browsers do not display the login dialog for cross-origin requests. To display the login dialog, GET request must be sent first. See below.
To display the login dialog for cross-origin requests, the browser must first send GET request. This request cannot be sent via XmlHttpRequest but only via directly accessing server, for example via iframe. The iframe onload event always fired after the user enters credentials to login the dialog. Only when iframe onload event fires the Ajax library can send requests. If the authentication fails onload event never fires.
Next Article:
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications You must be signed in to change notification settings
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement . We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Issues with Safari only - Origin is not allowed by Access-Control-Allow-Origin #595
hassant4 commented Nov 15, 2020
hassant4 commented Nov 15, 2020 • edited
Sorry, something went wrong.
- 👍 4 reactions
fedehuguet commented Sep 7, 2021
No branches or pull requests
WKWebView CORS Issue

- Skip to main content
- Select language
- Skip to search
- HTTP access control (CORS)
Preflighted requests and redirects
Credentialed requests and wildcards.
- Access-Control-Allow-Headers
- Access-Control-Request-Headers
Compatibility notes
A resource makes a cross-origin HTTP request when it requests a resource from a different domain, protocol, or port to its own. For example, an HTML page served from http://domain-a.com makes an <img> src request for http://domain-b.com/image.jpg. Many pages on the web today load resources like CSS stylesheets, images, and scripts from separate domains.
For security reasons, browsers restrict cross-origin HTTP requests initiated from within scripts. For example, XMLHttpRequest and Fetch follow the same-origin policy . So, a web application using XMLHttpRequest or Fetch could only make HTTP requests to its own domain. To improve web applications, developers asked browser vendors to allow cross-domain requests.

The Cross-Origin Resource Sharing ( CORS ) mechanism gives web servers cross-domain access controls, which enable secure cross-domain data transfers. Modern browsers use CORS in an API container - such as XMLHttpRequest or Fetch - to mitigate risks of cross-origin HTTP requests.
This article is for web administrators, server developers, and front-end developers. Modern browsers handle the client-side components of cross-origin sharing, including headers and policy enforcement. But this new standard means servers have to handle new request and response headers. Another article for server developers discussing cross-origin sharing from a server perspective (with PHP code snippets) is supplementary reading.
This cross-origin sharing standard is used to enable cross-site HTTP requests for:
- Invocations of the XMLHttpRequest or Fetch APIs in a cross-site manner, as discussed above.
- Web Fonts (for cross-domain font usage in @font-face within CSS), so that servers can deploy TrueType fonts that can only be cross-site loaded and used by web sites that are permitted to do so.
- WebGL textures .
- Images/video frames drawn to a canvas using drawImage .
- Stylesheets (for CSSOM access).
- Scripts (for unmuted exceptions).
This article is a general discussion of Cross-Origin Resource Sharing and includes a discussion of the necessary HTTP headers.
The Cross-Origin Resource Sharing standard works by adding new HTTP headers that allow servers to describe the set of origins that are permitted to read that information using a web browser. Additionally, for HTTP request methods that can cause side-effects on server's data (in particular, for HTTP methods other than GET , or for POST usage with certain MIME types ), the specification mandates that browsers "preflight" the request, soliciting supported methods from the server with an HTTP OPTIONS request method, and then, upon "approval" from the server, sending the actual request with the actual HTTP request method. Servers can also notify clients whether "credentials" (including Cookies and HTTP Authentication data) should be sent with requests.
Subsequent sections discuss scenarios, as well as provide a breakdown of the HTTP headers used.
Examples of access control scenarios
Here, we present three scenarios that illustrate how Cross-Origin Resource Sharing works. All of these examples use the XMLHttpRequest object, which can be used to make cross-site invocations in any supporting browser.
The JavaScript snippets included in these sections (and running instances of the server-code that correctly handles these cross-site requests) can be found "in action" at http://arunranga.com/examples/access-control/ , and will work in browsers that support cross-site XMLHttpRequest .
A discussion of Cross-Origin Resource Sharing from a server perspective (including PHP code snippets) can be found in the Server-Side Access Control (CORS) article.
Simple requests
Some requests don’t trigger a CORS preflight . Those are called “simple requests” in this article, though the Fetch spec (which defines CORS) doesn’t use that term. A request that doesn’t trigger a CORS preflight —a so-called “simple request”—is one that meets all the following conditions:
- Accept-Language
- Content-Language
- Content-Type (but note the additional requirements below)
- Viewport-Width
- application/x-www-form-urlencoded
- multipart/form-data
For example, suppose web content on domain http://foo.example wishes to invoke content on domain http://bar.other . Code of this sort might be used within JavaScript deployed on foo.example:
This will lead to a simple exchange between the client and the server, using CORS headers to handle the privileges:

Let us look at what the browser will send the server in this case, and let's see how the server responds:
Lines 1 - 10 are headers sent. The main HTTP request header of note here is the Origin header on line 10 above, which shows that the invocation is coming from content on the domain http://foo.example .
Lines 13 - 22 show the HTTP response from the server on domain http://bar.other . In response, the server sends back an Access-Control-Allow-Origin header, shown above in line 16. The use of the Origin header and of Access-Control-Allow-Origin show the access control protocol in its simplest use. In this case, the server responds with a Access-Control-Allow-Origin: * which means that the resource can be accessed by any domain in a cross-site manner. If the resource owners at http://bar.other wished to restrict access to the resource to requests only from http://foo.example , they would send back:
Access-Control-Allow-Origin: http://foo.example
Note that now, no domain other than http://foo.example (identified by the ORIGIN: header in the request, as in line 10 above) can access the resource in a cross-site manner. The Access-Control-Allow-Origin header should contain the value that was sent in the request's Origin header.
Preflighted requests
Unlike “simple requests” (discussed above) , "preflighted" requests first send an HTTP request by the OPTIONS method to the resource on the other domain, in order to determine whether the actual request is safe to send. Cross-site requests are preflighted like this since they may have implications to user data.
In particular, a request is preflighted if any of the following conditions is true:
The following is an example of a request that will be preflighted.
In the example above, line 3 creates an XML body to send with the POST request in line 8. Also, on line 9, a "customized" (non-standard) HTTP request header is set ( X-PINGOTHER: pingpong ). Such headers are not part of the HTTP/1.1 protocol, but are generally useful to web applications. Since the request uses a Content-Type of application/xml , and since a custom header is set, this request is preflighted.

Let's take a look at the full exchange between client and server. The first exchange is the preflight request/response :
Once the preflight request is complete, the real request is sent:
Lines 1 - 12 above represent the preflight request with the OPTIONS method. The browser determines that it needs to send this based on the request parameters that the JavaScript code snippet above was using, so that the server can respond whether it is acceptable to send the request with the actual request parameters. OPTIONS is an HTTP/1.1 method that is used to determine further information from servers, and is a safe method, meaning that it can't be used to change the resource. Note that along with the OPTIONS request, two other request headers are sent (lines 10 and 11 respectively):
The Access-Control-Request-Method header notifies the server as part of a preflight request that when the actual request is sent, it will be sent with a POST request method. The Access-Control-Request-Headers header notifies the server that when the actual request is sent, it will be sent with a X-PINGOTHER and Content-Type custom headers. The server now has an opportunity to determine whether it wishes to accept a request under these circumstances.
Lines 14 - 26 above are the response that the server sends back indicating that the request method ( POST ) and request headers ( X-PINGOTHER ) are acceptable. In particular, let's look at lines 17-20:
The server responds with Access-Control-Allow-Methods and says that POST , GET , and OPTIONS are viable methods to query the resource in question. Note that this header is similar to the Allow response header, but used strictly within the context of access control.
The server also sends Access-Control-Allow-Headers with a value of " X-PINGOTHER, Content-Type ", confirming that these are permitted headers to be used with the actual request. Like Access-Control-Allow-Methods , Access-Control-Allow-Headers is a comma separated list of acceptable headers.
Finally, Access-Control-Max-Age gives the value in seconds for how long the response to the preflight request can be cached for without sending another preflight request. In this case, 86400 seconds is 24 hours. Note that each browser has a maximum internal value that takes precedence when the Access-Control-Max-Age is greater.
Most browsers currently don’t support following redirects for preflighted requests. If a redirect occurs for a preflighted request, most current browsers will report an error message such as the following.
The request was redirected to 'https://example.com/foo', which is disallowed for cross-origin requests that require preflight
Request requires preflight, which is disallowed to follow cross-origin redirect
The CORS protocol originally required that behavior but was subsquently changed to no longer require it . However, most browsers have not yet implemented the change and still exhibit the behavior that was originally required.
So until browsers catch up with the spec, you may be able to work around this limitation by doing one or both of the following:
- change the server-side behavior to avoid the preflight and/or to avoid the redirect—if you have control over the server the request is being made to
- change the request such that it is a simple request that doesn’t cause a preflight
But if it’s not possible to make those changes, then another way that may be possible is to this:
- Make a simple request to determine (using Response.url for the Fetch API, or XHR.responseURL to determine what URL the real preflighted request would end up at).
- Make another request (the “real” request) using the URL you obtained from Response.url or XMLHttpRequest.responseURL in the first step.
However, if the request is one that triggers a preflight due to the presence of the `Authorization` header in the request, you won’t be able to work around the limitation using the steps above. And you won’t be able to work around it at all unless you have control over the server the request is being made to.
Requests with credentials
The most interesting capability exposed by both XMLHttpRequest or Fetch and CORS is the ability to make "credentialed" requests that are aware of HTTP cookies and HTTP Authentication information. By default, in cross-site XMLHttpRequest or Fetch invocations, browsers will not send credentials. A specific flag has to be set on the XMLHttpRequest object or the Request constructor when it is invoked.
In this example, content originally loaded from http://foo.example makes a simple GET request to a resource on http://bar.other which sets Cookies. Content on foo.example might contain JavaScript like this:
Line 7 shows the flag on XMLHttpRequest that has to be set in order to make the invocation with Cookies, namely the withCredentials boolean value. By default, the invocation is made without Cookies. Since this is a simple GET request, it is not preflighted, but the browser will reject any response that does not have the Access-Control-Allow-Credentials : true header, and not make the response available to the invoking web content.

Here is a sample exchange between client and server:
Although line 11 contains the Cookie destined for the content on http://bar.other , if bar.other did not respond with an Access-Control-Allow-Credentials : true (line 19) the response would be ignored and not made available to web content.
When responding to a credentialed request, the server must specify an origin in the value of the Access-Control-Allow-Origin header, instead of specifying the " * " wildcard.
Because the request headers in the above example include a Cookie header, the request would fail if the value of the Access-Control-Allow-Origin header were "*". But it does not fail: Because the value of the Access-Control-Allow-Origin header is " http://foo.example " (an actual origin) rather than the " * " wildcard, the credential-cognizant content is returned to the invoking web content.
Note that the Set-Cookie response header in the example above also sets a further cookie. In case of failure, an exception—depending on the API used—is raised.
The HTTP response headers
This section lists the HTTP response headers that servers send back for access control requests as defined by the Cross-Origin Resource Sharing specification. The previous section gives an overview of these in action.
- Access-Control-Allow-Origin
A returned resource may have one Access-Control-Allow-Origin header, with the following syntax:
The origin parameter specifies a URI that may access the resource. The browser must enforce this. For requests without credentials, the server may specify "*" as a wildcard, thereby allowing any origin to access the resource.
For example, to allow http://mozilla.org to access the resource, you can specify:
If the server specifies an origin host rather than "*", then it could also include Origin in the Vary response header to indicate to clients that server responses will differ based on the value of the Origin request header.
- Access-Control-Expose-Headers
The Access-Control-Expose-Headers header lets a server whitelist headers that browsers are allowed to access. For example:
This allows the X-My-Custom-Header and X-Another-Custom-Header headers to be exposed to the browser.
- Access-Control-Max-Age
The Access-Control-Max-Age header indicates how long the results of a preflight request can be cached. For an example of a preflight request, see the above examples.
The delta-seconds parameter indicates the number of seconds the results can be cached.
- Access-Control-Allow-Credentials
The Access-Control-Allow-Credentials header Indicates whether or not the response to the request can be exposed when the credentials flag is true. When used as part of a response to a preflight request, this indicates whether or not the actual request can be made using credentials. Note that simple GET requests are not preflighted, and so if a request is made for a resource with credentials, if this header is not returned with the resource, the response is ignored by the browser and not returned to web content.
Credentialed requests are discussed above.
- Access-Control-Allow-Methods
The Access-Control-Allow-Methods header specifies the method or methods allowed when accessing the resource. This is used in response to a preflight request. The conditions under which a request is preflighted are discussed above.
An example of a preflight request is given above , including an example which sends this header to the browser.
The Access-Control-Allow-Headers header is used in response to a preflight request to indicate which HTTP headers can be used when making the actual request.
The HTTP request headers
This section lists headers that clients may use when issuing HTTP requests in order to make use of the cross-origin sharing feature. Note that these headers are set for you when making invocations to servers. Developers using cross-site XMLHttpRequest capability do not have to set any cross-origin sharing request headers programmatically.
The Origin header indicates the origin of the cross-site access request or preflight request.
The origin is a URI indicating the server from which the request initiated. It does not include any path information, but only the server name.
Note that in any access control request, the Origin header is always sent.
- Access-Control-Request-Method
The Access-Control-Request-Method is used when issuing a preflight request to let the server know what HTTP method will be used when the actual request is made.
Examples of this usage can be found above.
The Access-Control-Request-Headers header is used when issuing a preflight request to let the server know what HTTP headers will be used when the actual request is made.
Examples of this usage can be found above .
Specifications
Browser compatibility.
The compatibility table in this page is generated from structured data. If you'd like to contribute to the data, please check out https://github.com/mdn/browser-compat-data and send us a pull request.
- Internet Explorer 8 and 9 expose CORS via the XDomainRequest object, but have a full implementation in IE 10.
- While Firefox 3.5 introduced support for cross-site XMLHttpRequests and Web Fonts, certain requests were limited until later versions. Specifically, Firefox 7 introduced the ability for cross-site HTTP requests for WebGL Textures, and Firefox 9 added support for Images drawn on a canvas using drawImage .
- Code Samples Showing XMLHttpRequest and Cross-Origin Resource Sharing
- Cross-Origin Resource Sharing From a Server-Side Perspective (PHP, etc.)
- Cross-Origin Resource Sharing specification
- XMLHttpRequest
- Using CORS with All (Modern) Browsers
- Using CORS - HTML5 Rocks
Document Tags and Contributors
- Same-origin policy
- Identifying resources on the Web
- Introduction to MIME Types
- Complete list of MIME Types
- Choosing between www and non-www URLs
- Overview of HTTP
- Evolution of HTTP
- HTTP Messages
- A typical HTTP session
- Connection management in HTTP/1.x
- Content Security Policy (CSP)
- HTTP Public Key Pinning (HPKP)
- HTTP Strict Transport Security (HSTS)
- Cookie security
- X-Content-Type-Options
- X-Frame-Options
- X-XSS-Protection
- Mozilla web security guidelines
- Mozilla Observatory
- HTTP authentication
- HTTP caching
- HTTP compression
- HTTP conditional requests
- HTTP content negotiation
- HTTP cookies
- HTTP range requests
- HTTP redirects
- HTTP specifications
- References:
- Accept-Charset
- Accept-Encoding
- Accept-Ranges
- Authorization
- Cache-Control
- Content-Disposition
- Content-Encoding
- Content-Length
- Content-Location
- Content-Range
- Content-Security-Policy
- Content-Security-Policy-Report-Only
- Content-Type
- If-Modified-Since
- If-None-Match
- If-Unmodified-Since
- Large-Allocation
- Last-Modified
- Proxy-Authenticate
- Proxy-Authorization
- Public-Key-Pins
- Public-Key-Pins-Report-Only
- Referrer-Policy
- Retry-After
- Set-Cookie2
- Strict-Transport-Security
- Transfer-Encoding
- Upgrade-Insecure-Requests
- WWW-Authenticate
- X-DNS-Prefetch-Control
- X-Forwarded-For
- X-Forwarded-Host
- X-Forwarded-Proto
- , <iframe> or <object> . Sites can use this to avoid clickjacking attacks, by ensuring that their content is not embedded into other sites."> X-Frame-Options
- 100 Continue
- 101 Switching Protocol
- 201 Created
- 202 Accepted
- 203 Non-Authoritative Information
- 204 No Content
- 205 Reset Content
- 206 Partial Content
- 300 Multiple Choices
- 301 Moved Permanently
- 303 See Other
- 304 Not Modified
- 307 Temporary Redirect
- 308 Permanent Redirect
- 400 Bad Request
- 401 Unauthorized
- 403 Forbidden
- 404 Not Found
- 405 Method Not Allowed
- 406 Not Acceptable
- 407 Proxy Authentication Required
- 408 Request Timeout
- 409 Conflict
- 411 Length Required
- 412 Precondition Failed
- 413 Payload Too Large
- 414 URI Too Long
- 415 Unsupported Media Type
- 416 Range Not Satisfiable
- 417 Expectation Failed
- 426 Upgrade Required
- 428 Precondition Required
- 429 Too Many Requests
- 431 Request Header Fields Too Large
- 451 Unavailable For Legal Reasons
- 500 Internal Server Error
- 501 Not Implemented
- 502 Bad Gateway
- 503 Service Unavailable
- 504 Gateway Timeout
- 505 HTTP Version Not Supported
- 511 Network Authentication Required
- element. If this value is absent, then any URI is allowed. If this directive is absent, the user agent will use the value in the <base> element."> CSP: base-uri
- CSP: block-all-mixed-content
- and <iframe>. For workers, non-compliant requests are treated as fatal network errors by the user agent."> CSP: child-src
- CSP: connect-src
- CSP: default-src
- CSP: font-src
- CSP: form-action
- , <iframe>, <object>, <embed>, or <applet>."> CSP: frame-ancestors
- and <iframe>."> CSP: frame-src
- CSP: img-src
- CSP: manifest-src
- and <video> elements."> CSP: media-src
- , <embed>, and <applet> elements."> CSP: object-src
- CSP: plugin-types
- CSP: referrer
- CSP: report-uri
- CSP: require-sri-for
- sandbox attribute. It applies restrictions to a page's actions including preventing popups, preventing the execution of plugins and scripts, and enforcing a same-origin policy."> CSP: sandbox
- elements, but also things like inline script event handlers (onclick) and XSLT stylesheets which can trigger script execution."> CSP: script-src
- CSP: style-src
- CSP: upgrade-insecure-requests
- CSP: worker-src
还能设置多个 Access-Control-Allow-Origin ?

Access-Control-Allow-Origin 是 HTTP 头部的一部分,用于实现跨域资源共享(Cross-Origin Resource Sharing,简称 CORS)。当一个网页尝试从与自身来源不同(即跨域)的服务器上获取资源时,浏览器会实施同源策略,阻止这种请求,除非服务器明确许可这种跨域访问。 Access-Control-Allow-Origin 头就是服务器用来告知浏览器哪些网站可以访问其资源的一种方式。
如果你希望只允许特定的源访问资源,可以在服务器端响应中设置 Access-Control-Allow-Origin 头,指定允许的源域名:
这表示只有来自 https://example.com 的网页可以成功请求此服务器上的资源。
如果你想允许任何源访问资源(注意这样做可能会带来安全风险),可以设置 Access-Control-Allow-Origin 为通配符 * :
在某些情况下,你可能需要根据请求的来源动态设置这个头部。以下是一个简单的示例,展示了如何在 Node.js 的 Express 应用中动态设置 Access-Control-Allow-Origin :
假设你有一个 API 服务器托管在 https://api.example.com ,并且你想让来自 https://myapp.com 的网页能够调用这个 API。
在 https://api.example.com 的服务器端,你需要设置响应头来允许来自 https://myapp.com 的跨域请求:
这样配置后, https://myapp.com 上的网页就可以成功请求 https://api.example.com/api/data 的资源了。
本文分享自 前端黑板报 微信公众号, 前往查看
如有侵权,请联系 [email protected] 删除。
本文参与 腾讯云自媒体分享计划 ,欢迎热爱写作的你一起参与!
Copyright © 2013 - 2024 Tencent Cloud. All Rights Reserved. 腾讯云 版权所有
深圳市腾讯计算机系统有限公司 ICP备案/许可证号: 粤B2-20090059 深公网安备号 44030502008569
腾讯云计算(北京)有限责任公司 京ICP证150476号 | 京ICP备11018762号 | 京公网安备号11010802020287
Copyright © 2013 - 2024 Tencent Cloud.
All Rights Reserved. 腾讯云 版权所有

IMAGES
VIDEO
COMMENTS
No 'Access-Control-Allow-Origin' header is present on the requested resource in NodeJS Hot Network Questions Factor from numeric vector drops every 100.000th element from its levels
The server responds with Access-Control-Allow-Origin: https://foo.example, restricting access to the requesting origin domain only.It also responds with Access-Control-Allow-Methods, which says that POST and GET are valid methods to query the resource in question (this header is similar to the Allow response header, but used strictly within the context of access control).
Note: null should not be used: "It may seem safe to return Access-Control-Allow-Origin: "null", but the serialization of the Origin of any resource that uses a non-hierarchical scheme (such as data: or file:) and sandboxed documents is defined to be "null".Many User Agents will grant such documents access to a response with an Access-Control-Allow-Origin: "null" header, and any origin can ...
The Access-Control-Allow-Origin response header is perhaps the most important HTTP header set by the CORS mechanism. The value of this header consists of origins that are allowed to access the resources. If this header is not present in the response headers, it means that CORS has not been set up on the server.
What is the Access-Control-Allow-Origin header? Access-Control-Allow-Origin is a CORS header. CORS, or Cross Origin Resource Sharing, is a mechanism for browsers to let a site running at origin A to request resources from origin B.
The 'Access-Control-Allow-Origin' header plays a pivotal role in CORS by indicating which origins are permitted to access the resources on the server. To send an OPTIONS request to a server using CURL, we can use the following command: -X 'OPTIONS' \. -H 'authority: sessions.bugsnag.com' \. -H 'accept: */*' \.
CORS and caching. If the server specifies an origin host rather than " * ", then it must also include Origin in the Vary response header to indicate to clients that server responses will differ based on the value of the Origin request header. Access-Control-Allow-Origin: https://developer.mozilla.org. Vary: Origin.
When responding to a credentialed request, the server must specify an origin in the value of the Access-Control-Allow-Origin header, instead of specifying the "*" wildcard. Option 1. As for Option 1, it was very insecure before major browsers changed the SameSite default value to Lax (and many browsers still haven't made that change).
Internet Explorer 9 and earlier ignores Access-Control-Allow headers and by default prohibits cross-origin requests for Internet Zone. To enable cross-origin access go to Tools->Internet Options->Security tab, click on "Custom Level" button. Find the Miscellaneous -> Access data sources across domains setting and select "Enable" option.
[Error] Failed to load resource: Origin https://www.rrrroll.com is not allowed by Access-Control-Allow-Origin. (upload, line 0) Having CORS issues with Safari only - Firefox, Chrome OK.
It affects only POST requests (GET and OPTIONS works fine) on Safari over HTTPS. [Error] Origin [origin] is not allowed by Access-Control-Allow-Origin. [Error] Failed to load resource: Origin [origin] is not allowed by Access-Control-Allow-Origin. [Error] XMLHttpRequest cannot load [apiURL] due to access control checks.
The response to the CORS request is missing the required Access-Control-Allow-Origin header, which is used to determine whether or not the resource can be accessed by content operating within the current origin.. If the server is under your control, add the origin of the requesting site to the set of domains permitted access by adding it to the Access-Control-Allow-Origin header's value.
The first thing we need is a server that's configured to host images with the Access-Control-Allow-Origin header configured to permit cross-origin access to image files.. Let's assume we're serving our site using Apache.Consider the HTML5 Boilerplate Apache server configuration file for CORS images, shown below:
There is an article related to CORS problems in WKWebView, but this forum does not allow me to include URL to it. So you can find it yourself at breautek.com, it it the only one published there. TL;DR: Setting 'Access-Control-Allow-Origin' to '*' is not enough, you should set it to the same value as the request 'Origin' header. 0. . Developer ...
Note that in any access control request, the Origin header is always sent.. Access-Control-Request-Method. The Access-Control-Request-Method is used when issuing a preflight request to let the server know what HTTP method will be used when the actual request is made.. Access-Control-Request-Method: <method> Examples of this usage can be found above.. Access-Control-Request-Headers
Safari 解决跨域问题:Access-Control-Allow-Origin. 一直以为safari很垃圾,没想到这么人性。 ... 浏览器的同源策略导致的,在服务器端添加 服务器收到预检请求后,检查了Origin、Access-Control-R...
I've also tried Access-Control-Allow-Origin: * and Safari also fails. I thought it might be the 3rd party cookies setting in Safari as our login server is on a different subdomain which sets the auth cookie but not sure how to check if that is actually the issue. Our apis are also on another subdomain and its the call to the apis that is not ...
Access-Control-Allow-Origin 头就是服务器用来告知浏览器哪些网站可以访问其资源的一种方式。. 如果你希望只允许特定的源访问资源,可以在服务器端响应中设置 Access-Control-Allow-Origin 头,指定允许的源域名:. 这表示只有来自 https://example.com 的网页可以成功请求此 ...
The Access-Control-Allow-Headers response header is used in response to a preflight request which includes the Access-Control-Request-Headers to indicate which HTTP headers can be used during the actual request. This header is required if the request has an Access-Control-Request-Headers header. Note: CORS-safelisted request headers are always ...
Access-Control-Allow-Origin in Safari. Ask Question Asked 9 years, 11 months ago. Modified 7 years, 1 month ago. Viewed 2k times ... Allow-Control-Allow-Origin and keep it enabled whenever you are working with requests. In case sometime it crashes, disable/re-enable it and it works. Share. Improve this answer. Follow